Grammarly Engineering Blog
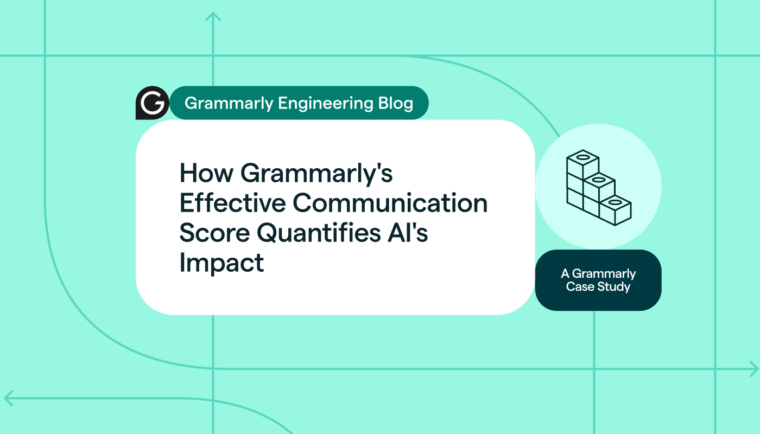
Featured
- ProductHow We Reduced Text Input Lag to Improve Web Performance: A Grammarly Case Study
- TeamEmpowering Women in Tech Through Mentorship at Grammarly
- InfrastructureEffective Customer Data Protection: How Grammarly Scales and Automates Our Vulnerability Management Program
- NLP/MLDetecting Delicate Text: Going Beyond Toxicity
DataThe Causal Effects of AI at Work
NLP/MLOne Model to Rule Them All: Our Path to Efficient On-Device Writing Assistance
DataHow Grammarly Built BEAM, an ML-Driven Budget Allocation Tool That Maximizes Marketing ROI
NLP/MLOn-Device AI at Scale: Grammarly’s Journey to Faster, More Reliable Models
ProductHow We Reduced Text Input Lag to Improve Web Performance: A Grammarly Case Study
NLP/MLAdvancing AI-Powered Intelligent Writing Assistance across Multiple Languages
ProductDemystifying Figma’s Variable Mode Inheritance
NLP/MLUnlocking Personalization With an On-Device Model for the Grammarly Keyboard
InfrastructureMastering the Art of Annotation
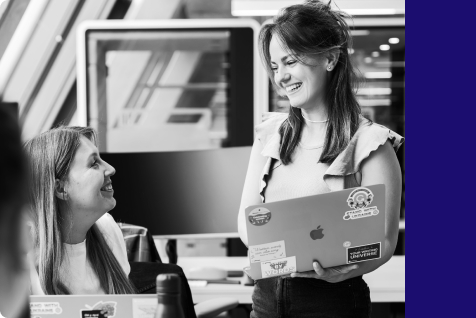
Shape the way millions of people communicate every day!